IPN (Webhook) Setup
To set up IPNs (also known as webhooks), you first set up the server and URL on your side, and then configure the IPN settings in your BlueSnap account. Once setup is complete, you may want to run a test to simulate an IPN.
IPN Types and Parameters
IPNs are sent as POST requests that contain parameters with data about the event.
For a list of available IPNs, refer to the IPN Name Reference.
For descriptions of all parameters that can be sent in an IPN, see IPN parameter reference.
1. Setting up your server to receive IPNs
To receive and process BlueSnap IPNs, you must set up your server and provide a URL where the IPNs can be sent.
SSL is required. Ensure that your server is configured to support HTTPS, with a valid server certificate.
IPN Request-Response Flow
Request: Your server should listen for the IPN messages from BlueSnap. These are typically sent in real-time with each event. The request will have a Content-type
of "application/x-www-form-urlencoded"
.
Response: Once you receive the IPN message, you should send an empty HTTP 200 response to BlueSnap.
If BlueSnap does not receive a confirmation that you received the IPN, we will make up to 11 attempts to resend it.
IP addresses used to send notifications
The IPNs are sent only from these BlueSnap IP addresses, so you may want to authorize these IPs.
209.128.93.254
209.128.93.98
141.226.140.100
141.226.141.100
141.226.142.100
141.226.143.100
209.128.93.232
141.226.140.200
141.226.141.200
141.226.142.200
141.226.143.200
Code Samples
The following JSP examples show how a merchant can capture and log the IPN data sent from BlueSnap. These IPN receiver examples check whether the IPN comes from BlueSnap servers, decode and parse the IPN, and then print the IPN to a log. There is a separate IPN receiver example for Production and Sandbox, with the relevant IP addresses per environment.
<%@page import="java.util.Arrays"%>
<%@page import="java.util.HashSet"%>
<%@page import="java.util.Set"%>
<%@page import="java.net.URLEncoder"%>
<%@page import="java.util.Map"%>
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%>
<%
String ipAddress = request.getRemoteAddr();
Set blueSnapIps = new HashSet(Arrays.asList("62.216.234.216, 209.128.93.254, 209.128.93.98, 38.99.111.60, 38.99.111.160, ..."));
if (blueSnapIps.contains(ipAddress)) {
Map parameters = request.getParameterMap();
for (String parameter : parameters.keySet()) {
System.out.println("[" + parameter + "=" + java.net.URLDecoder.decode(parameters.get(parameter)[0], "UTF-8") + "]");
}
}
else {
System.out.println(ipAddress + " is not a BlueSnap server!!!");
}
%>
<%@page import="java.util.Arrays"%>
<%@page import="java.util.HashSet"%>
<%@page import="java.util.Set"%>
<%@page import="java.net.URLEncoder"%>
<%@page import="java.util.Map"%>
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%>
<%
String ipAddress = request.getRemoteAddr();
Set<String> blueSnapIps = new HashSet<String>(Arrays.asList("209.128.93.232, 62.216.234.196. 38.99.111.50, 38.99.111.150, ..."));
if (blueSnapIps.contains(ipAddress)) {
Map<String, String[]> parameters = request.getParameterMap();
for (String parameter : parameters.keySet()) {
System.out.println("[" + parameter + "=" + java.net.URLDecoder.decode(parameters.get(parameter)[0], "UTF-8") + "]");
}
}
else {
System.out.println(ipAddress + " is not a BlueSnap server!!!");
}
%>
2. Enabling IPNs in BlueSnap
Using secure URLs
IPNs are transmitted via an HTTPS POST request to a unique URL on your site's server, which you define. Each URL must use the HTTPS protocol or the URL is not accepted.
We recommend that you enable IPNs for your entire BlueSnap account, which applies your IPN settings to all contracts in your account.
You can then define a different IPN URL for a specific contract, if needed.
Configuring your account IPN settings
The following steps describe how to enable IPNs for your account and add the IPN URLs to your account settings. Once this is complete, BlueSnap begins sending IPNs to your URLs in real-time.
-
In the Merchant Portal, go to Settings > IPN Settings.
-
Select the Receive Instant Payment Notifications check box. If you ever want to pause IPNs for your account, select Pause IPN Sending (only available through admin accounts).
-
In the IPN URL(s) field, enter the SSL enabled URL where you want to receive the IPNs from BlueSnap. All enabled IPNs are sent to this URL. Once you have entered your URL, you can test it by clicking the Test URL button to the right of the entry field.
-
(Optional) If you want to authenticate that an IPN originated from BlueSnap, you can add an encryption key by using the Generate Key button to create one or by entering your own key in the field. You can use this feature to validate the authenticity of the message.
- Using this feature adds two custom headers,
bls-signature
andbls-ipn-timestamp
, to your IPN requests. - The value of
bls-signature
is the signature of thebls-timestamp
concatenated with the IPN body in HMAC-SHA-256 using the encryption key. - You can compute the hashed output (
bls-signature
header value) of your secret key with an online SHA-256 tool (such as HMAC-SHA256 Hash Generator.- Enter
bls-ipn-timestamp
concatenated with the IPN body. The timestamp should be first and there should be no spaces between the timestamp and the start of the IPN body. - Enter the generated secret key.
- Click Compute Hash and validate against the received IPN header to confirm the hash output is the same as in
bls-signature
- Enter
- Using this feature adds two custom headers,
Enabling IPNs
-
To enable specific IPNs, click Select IPNs .
-
In the section that opens, toggle the button next to each IPN type that you want to receive. Be sure to click Submit in order to save your changes.
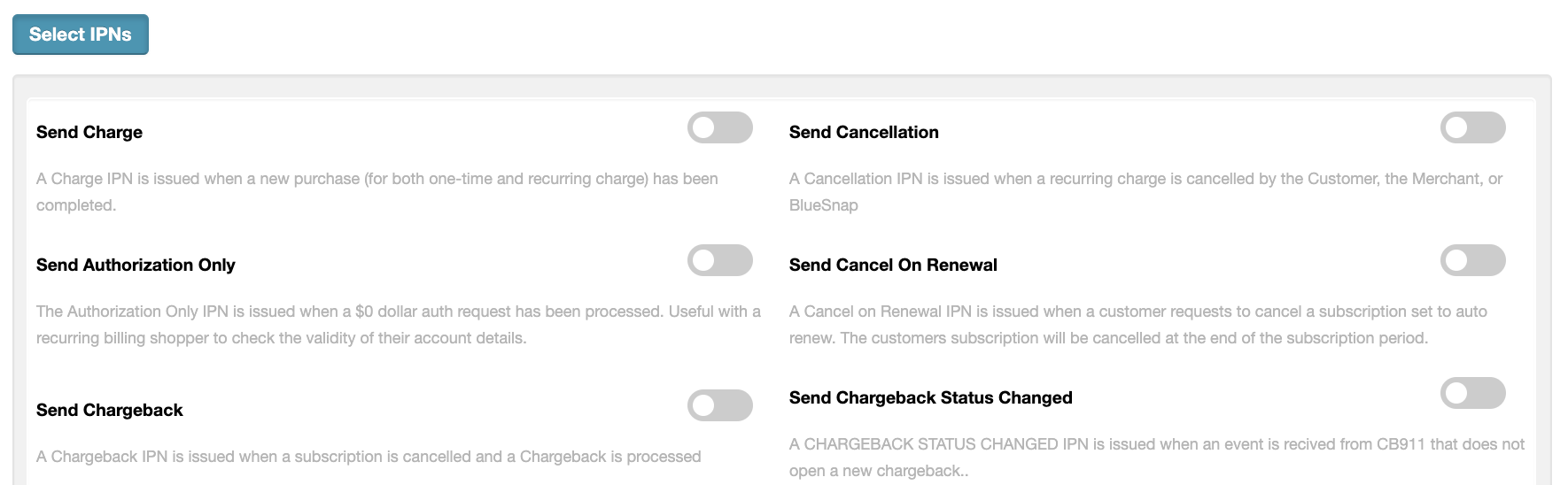
- To have IPNs sent to an additional URL, click Add IPN URL, and then enter the additional URL where you want to receive the IPNs from BlueSnap.
- If you want to have any IPNs sent to this additional URL, toggle the button next to each IPN type that you want to receive. Note that any IPN types you select here are also sent to your main IPN URL. Click Submit.
- When setting up Instant Notifications as a merchant, you can select the Require Notification Receipt option to obtain confirmation that the incoming notification has been sent from BlueSnap. This authentication process requires you to complete a number of set-up steps, and you should ONLY select this option when you are ready to complete the process; otherwise, you might begin receiving automated notification failure messages.
Note
If you have not already done so, you must set up a Data Protection Key
Your Instant notifications process must be able to handle the encrypted attributeauthKey
and set a response ofOK + Data Protection Key
encrypted in MD5 Hex.
Once this option is enabled, BlueSnap treats each IPN that does not get an encrypted response as a failure and attempts to resend it up to five more times.
Configuring a separate IPN URL for a specific contract
Follow these steps to define a different IPN URL for a specific contract. This overrides the global IPN URL defined for the account.
-
In the Merchant Portal, go to Product Catalog > Products in the left menu.
-
Expand the relevant product to view its contracts, and then click the name of the contract where you want to set up an IPN.
-
In the Notifications section, select Receive Instant Payment Notifications check box.
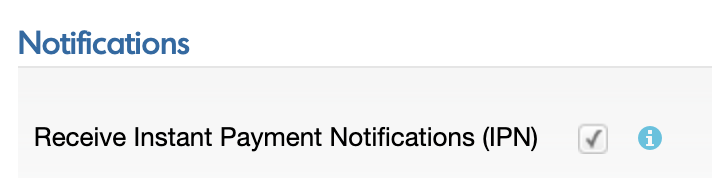
-
Select the checkbox next to each IPN type that you want to receive.
-
Select Use a specific IPN URL for this contract and enter the URL(s) where IPNs for this contract should be sent.
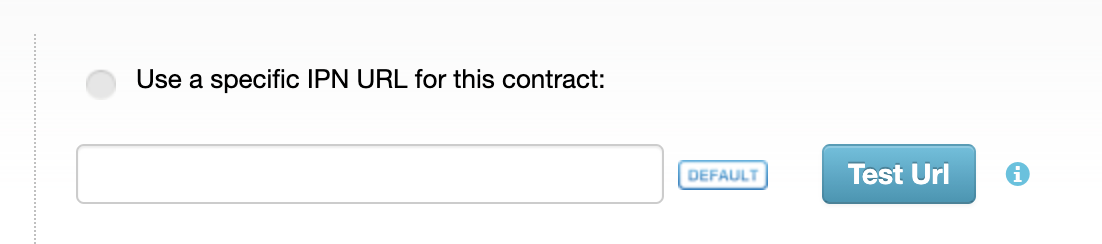
- To have the IPNs sent to an additional URL, click Add IPN URL, and then enter the additional URL where you want to receive the IPNs from BlueSnap.
- If you would like to have any IPNs sent to this additional URL, select the checkbox next to each IPN type that you want to receive. Note that any IPN types you select here are also sent to your main IPN URL. Click Submit.
3. Testing IPNs
Before you go live, we recommend testing your IPNs and ensure you properly receive, process, and respond to the notifications.
Troubleshooting
If you have set up your IPN URL but you do not receive an IPN, you can follow these troubleshooting steps:
- Resend the IPN: In the Merchant Portal, click Find a Transaction, then go to one of your existing order records. In the Resend Menu, select IPN, and click Send.
-
Verify that the IPN URL is set up at both the account and contract level: Check the account-level IPN settings under Settings > IPN Settings. In some cases, you may have different contract IPN settings, which overrides your account-level IPN settings. Check the contract-level IPN settings on the General contract settings page, under Notifications.
-
Try simulating an IPN, as described below.
-
If you still don’t receive IPNs, contact Merchant Support.
Simulating an IPN
There are two ways to simulate an IPN so you can verify that you are receiving the IPNs and debug any potential issues.
Option 1
-
In the Merchant Portal, go to Settings > IPN Settings.
-
Using the IPN Simulator, select the IPN you want to test and enter the URL to use for testing.
-
Click Generate IPN.
-
The IPN Simulator generates the selected IPN and sends it to the URL entered. The parameters in each IPN are the same parameters used in production; however, the values returned are generic.
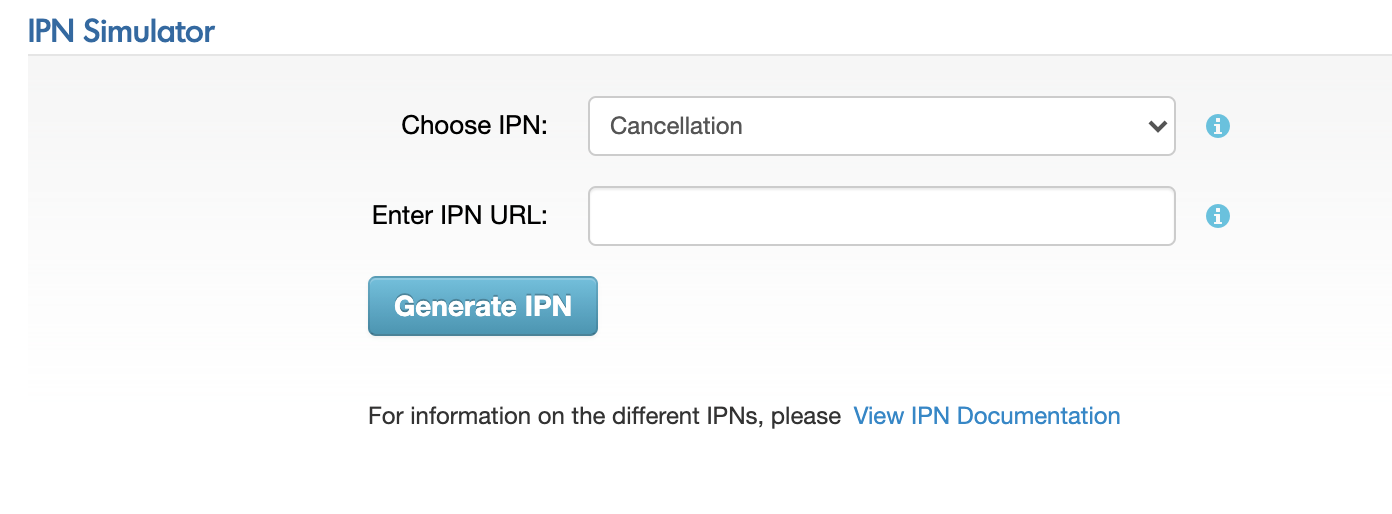
Option 2
-
In the Merchant Portal, go to Settings > General Settings.
-
Enter your email address in the Admin email address field so that you receive any emails about IPN errors, then click Submit.
-
Create a contract with a specific IPN URL (refer to Configuring a separate IPN URL for a specific contract). For this contract, enter an invalid IPN URL. This forces BlueSnap to send an IPN error email with a copy of the IPN.
-
Place a test order via the BuyNow checkout page for the contract you just set up.
When the order is complete, BlueSnap sends an IPN.
Because the IPN URL is invalid, BlueSnap copies the IPN to an email and sends it to the Admin email address you defined. You can then use this example IPN to parse each of the parameters and verify that they are configured correctly.
Updated 8 months ago