Implementing Hosted Pages
Before you Begin
Before you can implement BlueSnap Checkout Hosted Pages, complete the following and satisfy the Website Requirements:
- Create your API credentials.
- Assign a static IP address to your web server.
- Select a sandbox or production environment implementation.
Website Requirements
BlueSnap Checkout gives you the option to customize your checkout page. It also requires that you provide landing pages for your shoppers after checkout.
You can configure checkout page settings and landing page URLs on Checkout Page > Hosted Pages in your Merchant portal:
- Checkout page — Select features and choose colors for your checkout page to match your website.
- Success page — Post-checkout landing page that notifies your shopper that the transaction succeeded. For example, this page might summarize the payment and shipping information. Define this with Success URL in your Merchant portal.
- Cancel page — Post-checkout landing page that confirms that the transaction was canceled and no funds will be transferred from your shopper's account. Define this with Cancel URL in your Merchant portal.
For additional details, see Page Design and Custom Fields.
Basic Implementation
These steps implement BlueSnap Checkout so you can complete a sale on your website and verify the transaction.
After you complete the basic implementation, the subsequent sections describe optional setup, such as payment method requirements, iframe implementation, and returning shopper payment options.
Step 1: Add the BlueSnap Javascript
Add the BlueSnap Javascript to your site. BlueSnap provides a Javascript file for both the sandbox and production environments. Below are the script elements for each environment:
Sandbox script element
<script type="text/javascript" src="sandpay.bluesnap.com/web-sdk/5/bluesnap.js"></script>
Production script element
<script type="text/javascript" src="pay.bluesnap.com/web-sdk/5/bluesnap.js"></script>
Step 2: Create a JWT Token
When your shopper is ready to complete their purchase, you must create a JSON Web Token (JWT) for the checkout process with BlueSnap. The JWT securely stores data about the transaction and allows BlueSnap to verify your identity. This token also prevents unauthorized websites from creating BlueSnap checkout pages on your behalf.
To create a BlueSnap Checkout JWT, complete the following:
-
Send a request for the JWT from your client-side application to its web server.
-
Send a server-to-server request to the
/services/2/bn3-services/jwt
endpoint in your BlueSnap environment.
BlueSnap provides secure payment parameters that you can include in the request body. The following examples request a JWT both transaction and subscription purchases in a sandbox environment. Replace the Authorization header valueYOUR_API_KEY
with your API credentials:curl -v -X POST https://sandbox.bluesnap.com/services/2/bn3-services/jwt \ -H 'Content-Type: application/json' \ -H 'Accept: application/json' \ -H 'Authorization: Basic YOUR_API_KEY' \ -d ' { "mode": "one_time", "onBehalf": "false", "currency": "USD", "successUrl": "http://www.example.com/success", "cancelUrl": "http://www.example.com/cancel", "merchantTransactionId": "myid1234", "lineItems": [ { "id": "001", "quantity": 2, "label": "T-Shirt", "description": "T-Shirt with logo", "amount": 20 }, { "id": "002", "quantity": 1, "label": "Shorts", "description": "Green shorts with pockets", "amount": 15 } ] }'
curl -v -X POST https://sandbox.bluesnap.com/services/2/bn3-services/jwt \ -H 'Content-Type: application/json' \ -H 'Accept: application/json' \ -H 'Authorization: Basic YOUR_API_KEY' \ -d ' { "mode": "subscription", "successUrl": "http://www.mySite.com/success", "cancelUrl": "http://www.mySite.com/cancel", "planItems": [ { "id": "001", // the plan id from createPlan API "quantity": 1, "label": "AAA subscription", // override the plan-name "recurringChargeAmount": 20, // override the recurringChargeAmount "trialPeriodDays": 14, // override the trialPeriodDays "initialChargeAmount": 10 // override the initialChargeAmount } ] }'
Note
The
successUrl
andcancelUrl
parameters override the Success URL and Cancel URL settings in your Merchant portal. Omit them in the request to use the URLs defined in your page design settings.
A successful request returns a response that includes your token in the jwt
property:
{
"jwt": "eyJhbGciOiJIUzI1NiJ9.eyJwYXlsb2FkIjp7ImNvbW1vbkp3dFBheWxvYWQiOnsiaWQiOiI1OTU4NTkxODYxNTgwMDg5OTI3NzEiLCJtZXJjaGFudElkIjo0MDAwNDgsImRhdGVDcmVhdGVkIjoxNjA2OTk2MjI5OTExLCJlbnYiOiJERVY2In0sImN1cnJlbmN5IjoiVVNEIiwic3VjY2Vzc1VybCI6Imh0dHBzOi8vaG9tZS5ibHVlc25hcC5jb20iLCJjYW5jZWxVcmwiOiJodHRwczovL2FwLmJsdWVzbmFwLmludDo0MDA0L2NhbmNlbCIsIm1lcmNoYW50VHJhbnNhY3Rpb25JZCI6IjU5NTg1OTE4NjE1ODAwODk5Mjc3MSIsImxpbmVJdGVtcyI6W3siaWQiOiJpZDEiLCJsYWJlbCI6IlJhaW5ib3ciLCJkZXNjcmlwdGlvbiI6IlRvdWNoIHRoZSBSYWluYm93IiwicXVhbnRpdHkiOjEsImFtb3VudCI6MTAuMCwiaW1nVXJsIjpudWxsfV19fQ.Mi4eiNKCsGDTsLHnTXPfe4-UNpQYSY-OvKufwZr2jR0",
"merchantTransactionId": "myid1234"
}
For details about this response object, see Create Token API.
Token Expiration
A JWT token expires if either of the following are true:
- The token was successfully used to processed a transaction.
- The token has expired. By default, the token expires two hours after you create it.
If the default expiration period is not sufficient, you can set a custom expiration period with the expH
payment parameter in the request body. This parameter accepts an integer that defines the number of hours until the JWT expires. For example, "exph": 10
means that the JWT expires 10 hours after it is created. This property accepts up to 8,760
hours (1 year). If you attempt to send this parameter in your request and it is not successful, you can speak with your implementation manager or contact merchant support for privileges to set a custom expiration period.
The following request snippet creates a JWT token that expires after 10 hours:
curl -v -X POST https://sandbox.bluesnap.com/services/2/bn3-services/jwt \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Authorization: Basic YOUR_API_KEY' \
-d '
{
"mode": "one_time",
"expH": 10,
...
}'
Step 3: Complete your Sale
Note
Some payment methods require additional implementation after this step. For details, see Adding Payment Methods.
Now that your server has a JWT token that contains your shopper's transaction information, you can initiate and display the BlueSnap checkout page to begin the checkout process. To display the checkout page for the transaction, your client-side application must retrieve the token from your server, then redirect your shopper to the BlueSnap checkout page along with the token.
The following code sample registers an event handler to a payment button. When the shopper clicks the button, the event fires and begins the checkout process with the following steps:
- Gets the token from your server and parses the response as JSON.
- Extracts the token from the parsed JSON and stores it in an sdkRequest object.
- Passes the
sdkRequest
object tobluesnap.redirectToPaymentPage
. This function redirects the shopper to the BlueSnap checkout page and includes the token that contains the transaction information.
document.getElementById('payButton').addEventListener('click', function () {
// 1. Get token from your server
fetch('/path/to/token')
.then((response) => response.json())
.then((data) => {
const token = data.token;
// 2. Store token in sdkRequest object
const sdkRequest = {
jwt: token
};
// 3. Redirect shopper to BlueSnap checkout page
bluesnap.redirectToPaymentPage(sdkRequest);
})
.catch((error) => {
console.log(error);
});
});
When your shopper completes the checkout process, BlueSnap processes the transaction and redirects your shopper to the successUrl
stored in the JWT token. BlueSnap appends transaction data to the URL with Return URL Parameters.
Step 4: Verify your Transaction
To ensure transactions are valid, we recommend that you compare and validate your transaction details against existing information. You can retrieve transaction details from the URL parameters appended to your successUrl
.
There are two ways to verify a transaction:
-
Send a Retrieve API request to get transaction details and confirm that the transaction succeeded. Because BlueSnap operates in two different data centers, you need to request the transaction from the data center that processed the transaction.
To determine which data center processed the transaction, use the BlueSnap
transactionId
property that was appended to thesuccessUrl
. The last digit in the invoice ID indicates the data center:transactionId Call Domain Even number ws1.bluesnap.com
Odd number ws1.bluesnap.com
-
Set up IPNs to receive an alert for any event, including a charge.
Payment Method Requirements
Note
If you want to accept Apple Pay, please contact Merchant Support to enable it on your account.
Some payment methods require that you display specific information in the successUrl
. To gather the additional information, use the transactionId
Return URL parameter to retrieve transaction details with the Payment API.
The following sections explain the Payment API requests and required information for each payment method.
SEPA Direct Debit
To complete implementation for SEPA Direct Debit:
- Retrieve the transaction details with the
transactionId
. - Complete the SEPA implementation process, starting with Step 3.
Pay by Bank
To complete implementation for Pay by Bank (Open Banking):
- Retrieve the transaction details with the
transactionId
. - Complete the steps in Pay by Bank Transaction Processing.
Embed in an iframe
Note
The following payment methods are not supported in iframe mode:
- ApplePay
- Pay by Bank
You can configure BlueSnap Checkout to open within an iframe, an HTML element that embeds an HTML page within an HTML page. Embedding an iframe means that you do not have to redirect your shopper during checkout.
To add the BlueSnap Checkout iframe, add the following <div>
element to your page:
<div data-bluesnap="iframe-container"></div>
Redirection
You can configure redirection with the displayData.iframeOptions.topRedirect
property in the sdkRequest object.
By default, topRedirect
is false
. This means that only the iframe window is redirected to your success or cancel URL. If you set topRedirect
to true
, BlueSnap Checkout redirects the entire page to the success or cancel URL.
Note
PayPal and Sofort always perform top redirection and ignore the
topRedirect
setting.
Returning Shoppers
When a transaction is successful, the object in the response of the Retrieve API request includes the shopper ID in the vaultedShopperId
property. If you want to allow returning shoppers to use their saved information for payments, you can save the vaultedShopperId
and associate it with your shopper. When the shopper checks out again and you are sending a token creation request, you can then include the vaultedShopperId
in the shopperId
field.
To leverage this feature, configure the checkout page to display a checkbox that the shopper can use to save their card information after a successful transaction. Here are some examples of how this checkout experience might look:
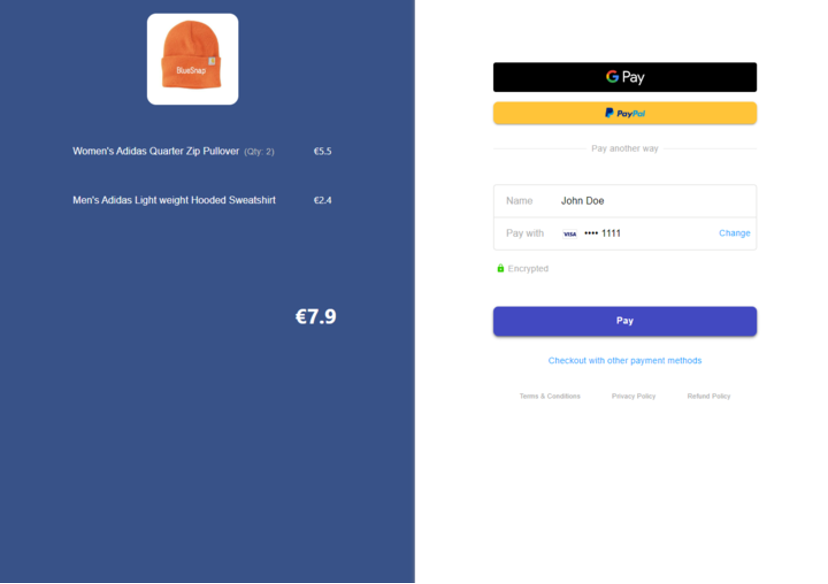
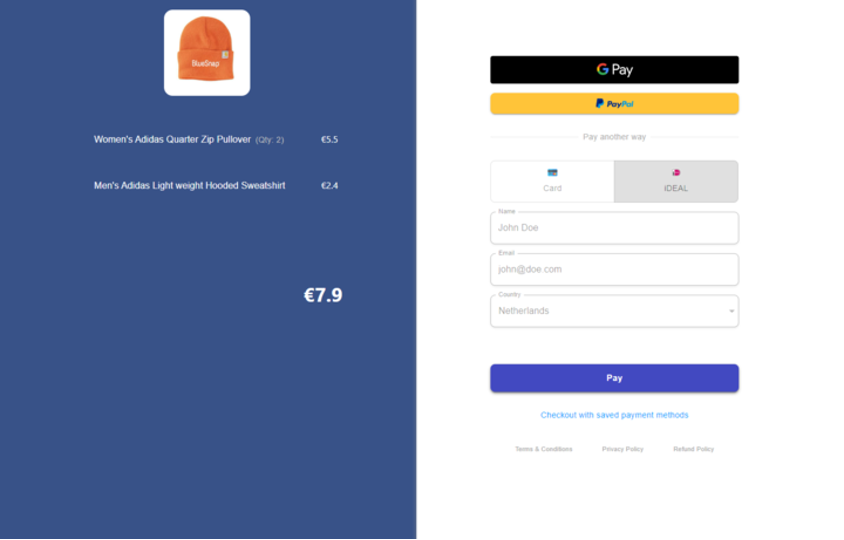
Next steps
After you finish implementing BlueSnap Checkout, explore the documentation for additional ways to get the most out of BlueSnap's platform.
Learn about BlueSnap's IPN (Webhooks) and how to stay informed of important events.
Updated about 2 months ago